Learning Objectives:
1. Create a table and update the table.
2. Query and scan the table.
3. Delete the table.
Step 1: Create an environment in Cloud9.
In AWS management console, search for Cloud9 service and click on create environment. Here we have created the environment in US East (N. Virginia) - us-east-1 region.
In case you want to use a different AWS region do make changes as shown at respective places in this tutorial.
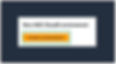
Give details:
Name: MyCloud9
Description: Cloud9 for DynamoDB
Keep the rest as default and click on Create.
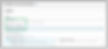
You can see the environment is created.
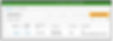
Step 2: Click on Open in Cloud9 IDE.
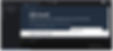
Step 3: In the terminal, run the following command:
pip install boto3

Step 4: In File, click on New From Template, select Python File.

Now save the file, with the name “create_table.py”

Step 5: Copy the below code to create a table.
Code
import logging import boto3 from botocore.exceptions import ClientError class MoviesTableCreator: def __init__(self, dynamodb_resource): self.dynamodb_resource = dynamodb_resource def create_table(self, table_name): try: table = self.dynamodb_resource.create_table( TableName=table_name, KeySchema=[ {'AttributeName': 'year', 'KeyType': 'HASH'}, # Partition key {'AttributeName': 'title', 'KeyType': 'RANGE'} # Sort key ], AttributeDefinitions=[ {'AttributeName': 'year', 'AttributeType': 'N'}, {'AttributeName': 'title', 'AttributeType': 'S'} ], ProvisionedThroughput={'ReadCapacityUnits': 10, 'WriteCapacityUnits': 10} ) table.wait_until_exists() except ClientError as e: if e.response['Error']['Code'] == 'ResourceInUseException': logging.info("Table %s already exists.", table_name) else: logging.error( "Couldn't create table %s. Error: %s", table_name, e.response['Error']['Message']) raise else: return table if __name__ == "__main__": # Initialize the DynamoDB resource dynamodb = boto3.resource('dynamodb') # Replace 'MyNewMoviesTable' with your desired, unique table name table_name = 'MyNewMoviesTable' # Create the DynamoDB table (if not already created) table_creator = MoviesTableCreator(dynamodb) created_table = table_creator.create_table(table_name) if created_table: print(f"Table '{table_name}' created: {created_table.table_status}")
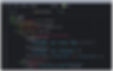
After copying the code save the file using Ctrl+S.
In the terminal execute the following command.
python create_table.py
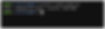
You can go to DynamoDB service and check your Table is created.

Step 6: Go back to Cloud9, similarly create a new file as mentioned in Step 4, and save the file with a name, “put_get_update.py”
Copy the following code to add an item and view the item. Replace the region ('us-east-1') with whatever region (‘xx-xxx-x’) you have selected.
import boto3
from decimal import Decimal
# Initialize the DynamoDB resource
dynamodb = boto3.resource('dynamodb', region_name='us-east-1')
# Access your DynamoDB table
table_name = 'MyNewMoviesTable' # Replace with your table name
table = dynamodb.Table(table_name)
# Define the movie item to put
movie_item = {
'year': 2023, # Replace with the desired year
'title': 'New Movie', # Replace with the movie title
'info': {
'director': 'Director Name',
'genre': 'Action',
'rating': Decimal('4.5') # Use Decimal for ratings
}
}
try:
response = table.put_item(Item=movie_item)
print("Movie item added successfully!")
except Exception as e:
print(f"Error adding movie item: {str(e)}")
# Define the key of the movie item to get
year_to_get = 2023 # Replace with the year of the movie you want to retrieve
title_to_get = 'New Movie' # Replace with the title of the movie you want to retrieve
try:
response = table.get_item(
Key={
'year': year_to_get,
'title': title_to_get
}
)
movie_item = response.get('Item')
if movie_item:
print("Movie item retrieved:", movie_item)
else:
print("Movie item not found.")
except Exception as e:
print(f"Error getting movie item: {str(e)}")
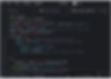
Save the file using Ctrl+S.
Next, in the terminal execute the following command.
python put_get_update.py

You can see the message as movie item created successfully and we have retrieved the data as well.
Verify the item added, go to DynamoDB console and in the Explore Items you will be able to see Item created.

Click on the item and you can see the information of the given item.
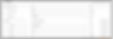
Now we will update the item. We will update the current item rating from 4.5 to 3.8.
Copy the below code and paste it in “put_get_update.py” file at end of the file.
Code
# Define the key of the movie item to update
year_to_update = 2023 # Replace with the year of the movie you want to update
title_to_update = 'New Movie' # Replace with the title of the movie you want to update
# Define the new rating and plot values
new_rating = Decimal('3.8') # Use Decimal for ratings
try:
response = table.update_item(
Key={
'year': year_to_update,
'title': title_to_update
},
UpdateExpression="set info.rating = :new_rating",
ExpressionAttributeValues={
':new_rating': new_rating
},
ReturnValues="ALL_NEW" # Modify this if you want different return values
)
updated_item = response.get('Attributes')
if updated_item:
print("Movie item updated successfully:", updated_item)
else:
print("Movie item not found or not updated.")
except Exception as e:
print(f"Error updating movie item: {str(e)}")

Save the file and in the terminal execute the file using the below command.
python put_get_update.py

You will get a message of Movie item updated successfully!
To check, go to DynamoDB console and click on item, you will be able to see the rating is changed from 4.5 to 3.8
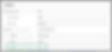
Step 7: Populate data in the table (“NewMovieTable”) using JSON file.
For this, create a new JSON file and name it as movies.json and paste the following code in this file-
Code
[ { "year": 2022, "title": "Movie 1", "info": { "director": "Director 1", "genre": "Action", "rating": 4.5 } }, { "year": 2023, "title": "Movie 2", "info": { "director": "Director 2", "genre": "Comedy", "rating": 3.8 } }, { "year": 2022, "title": "Movie 3", "info": { "director": "Director 3", "genre": "Drama", "rating": 4.0 } } ]
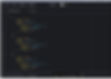
Save the file and create a new python file and name it as “add_item.py”
Paste the following code in this file. Replace the region ('us-east-1') with whatever region (‘xx-xxx-x’) you have selected.
Code
import json import boto3 from decimal import Decimal # Initialize the DynamoDB resource dynamodb = boto3.resource('dynamodb', region_name='us-east-1') # Access your DynamoDB table table_name = 'MyNewMoviesTable' # Replace with your table name table = dynamodb.Table(table_name) # Read movie data from the JSON file with open('movies.json', 'r') as json_file: movie_data = json.load(json_file) # Put each movie item into the table for movie in movie_data: try: response = table.put_item( Item={ 'year': movie['year'], 'title': movie['title'], 'info': { 'director': movie['info']['director'], 'genre': movie['info']['genre'], 'rating': Decimal(str(movie['info']['rating'])) } } ) print(f"Movie item added: {movie['title']} ({movie['year']})") except Exception as e: print(f"Error adding movie item: {str(e)}")
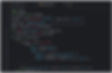
Save the file and execute the file in the terminal with the following command:
python add_item.py
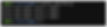
You can see the item is successfully added.
To verify, go to DynamoDB console and in the Explore Items you can see the items are added successfully.
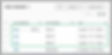
Step 8: Now we want to run a query for movies that were released in a given year.
For this, create a new python file and give name as “query.py”
Now paste the following code in this file. Replace the region ('us-east-1') with whatever region (‘xx-xxx-x’) you have selected.
Code
import boto3 # Initialize the DynamoDB resource dynamodb = boto3.resource('dynamodb', region_name='us-east-1') # Access your DynamoDB table table_name = 'MyNewMoviesTable' # Replace with your table name table = dynamodb.Table(table_name) # Define the year you want to query year_to_query = 2023 # Replace with the desired year try: response = table.query( KeyConditionExpression='#yr = :year', ExpressionAttributeNames={ '#yr': 'year' # Use a placeholder for the reserved keyword }, ExpressionAttributeValues={ ':year': year_to_query } ) items = response['Items'] if items: for item in items: print(item) else: print("No items found for the specified year.") except Exception as e: print(f"Error querying table: {str(e)}")
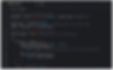
Save the file and execute the file using the following command in the terminal.
python query.py

As in the code, we have given year as 2023, so it is returning the movies released in the year 2023.
Step 9: Now we want to perform scan for movies that were released in a range of years.
Create a new python file, give a name as “scan.py” and paste the following code in this file.Replace the region ('us-east-1') with whatever region (‘xx-xxx-x’) you have selected.
import boto3
# Initialize the DynamoDB resource
dynamodb = boto3.resource('dynamodb', region_name='us-east-1')
# Access your DynamoDB table
table = dynamodb.Table('MyNewMoviesTable') # Replace 'YourTableName' with your actual table name
try:
response = table.scan()
items = response['Items']
for item in items:
print(item)
except Exception as e:
print(f"Error scanning the table: {str(e)}")
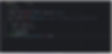
Save the file and execute the file in the terminal with the following command.
python scan.py

You can see the output as above.
Step 10: To perform delete an item from the table.
Create new file and name it as “delete_item.py” and paste the following code in this file.
import boto3
# Initialize the DynamoDB resource
dynamodb = boto3.resource('dynamodb')
# Access your DynamoDB table
table = dynamodb.Table('MyNewMoviesTable')
# Define the key of the item to delete (year and title)
year_to_delete = 2023
title_to_delete = 'Movie 2'
try:
response = table.delete_item(
Key={
'year': year_to_delete,
'title': title_to_delete
}
)
print(f"Item {year_to_delete}, {title_to_delete} deleted successfully!")
except Exception as e:
print(f"Error deleting item: {str(e)}")
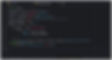
Save the file and execute the file in the terminal using following command.
python delete_item.py

To verify delete action, go to DynamoDB console.
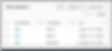
Step 11: Delete the table.
Create a new python file and name it as “delete_table.py” and paste the following code in this file.Replace the region ('us-east-1') with whatever region (‘xx-xxx-x’) you have selected.
import boto3
# Initialize the DynamoDB resource
dynamodb = boto3.resource('dynamodb', region_name='us-east-1')
# Replace 'YourTableName' with your actual table name
table_name = 'MyNewMoviesTable'
try:
# Access the table and delete it
table = dynamodb.Table(table_name)
table.delete()
print(f"Table '{table_name}' deleted successfully!")
except Exception as e:
print(f"Error deleting table: {str(e)}")

Save the file and execute the file in the terminal using following command.
python delete_table.py

Verify table deletion by going to DynamoDB console.
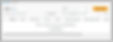
Note: Close the Cloud9 IDE and delete the environment if no longer needed.
Was this document helpful? How can we make this document better. Please provide your insights. You can download PDF version for reference.
For your aws certification needs or for aws learning contact us.