Revised Tutorial blog to create a Microservice using AWS Lambda & Amazon API Gateway
- Vaibhav Deshpande
- Nov 9, 2023
- 4 min read
Updated: Nov 27, 2023
Learning Objectives:
1. Learn to create a REST API using Amazon API Gateway.
2. Learn to create AWS Lambda function using AWS CLI.
Step 1: In AWS management console, go to IAM service.
1. Open the Policies page of the IAM console.
2. Choose Create Policy.
3. Choose the JSON tab, and then paste the following custom policy into the JSON editor.
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "Stmt1428341300017",
"Action": [
"dynamodb:DeleteItem",
"dynamodb:GetItem",
"dynamodb:PutItem",
"dynamodb:Query",
"dynamodb:Scan",
"dynamodb:UpdateItem"
],
"Effect": "Allow",
"Resource": "*"
},
{
"Sid": "",
"Resource": "*",
"Action": [
"logs:CreateLogGroup",
"logs:CreateLogStream",
"logs:PutLogEvents"
],
"Effect": "Allow"
}
]
}
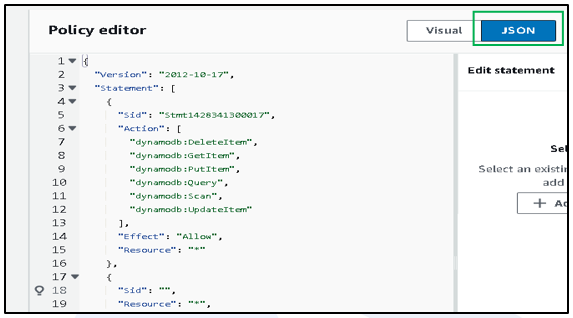
4. Policy name: lambda-apigateway-policy
5. Choose Create policy.
Step 2: In IAM console, choose Create role.
For the type of trusted entity, choose AWS service, then for the use case, choose Lambda.
Choose Next.
In the policy search box, enter lambda-apigateway-policy
In the search results, select the policy that you created (lambda-apigateway-policy), and then choose Next.
Under Role details, for the Role name, enter lambda-apigateway-role, then choose Create role.
You need the Amazon Resource Name (ARN) of the role you just created. On the Roles page of the IAM console, choose the name of your role (lambda-apigateway-role) and copy the Role ARN displayed on the Summary page.
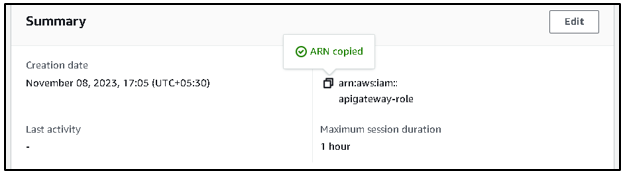
Step 3: In AWS management console, go to Cloud9 and create an environment with name as my-env.
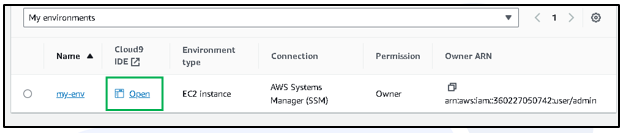
Create a new file and paste the below code in the file.
import boto3
import json
# define the DynamoDB table that Lambda will connect to
tableName = "lambda-apigateway"
# create the DynamoDB resource
dynamo = boto3.resource('dynamodb').Table(tableName)
print('Loading function')
def handler(event, context):
'''Provide an event that contains the following keys:
- operation: one of the operations in the operations dict below
- payload: a JSON object containing parameters to pass to the
operation being performed
'''
# define the functions used to perform the CRUD operations
def ddb_create(x):
dynamo.put_item(**x)
def ddb_read(x):
dynamo.get_item(**x)
def ddb_update(x):
dynamo.update_item(**x)
def ddb_delete(x):
dynamo.delete_item(**x)
def echo(x):
return x
operation = event['operation']
operations = {
'create': ddb_create,
'read': ddb_read,
'update': ddb_update,
'delete': ddb_delete,
'echo': echo,
}
if operation in operations:
return operations[operation](event.get('payload'))
else:
raise ValueError('Unrecognized operation "{}"'.format(operation))
Save the code as a file named LambdaFunctionOverHttps.py
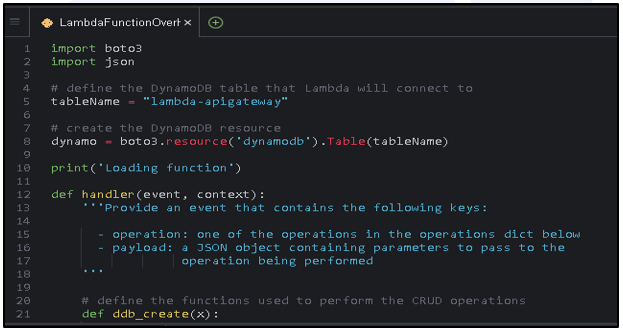
In the terminal, execute the following command.
zip function.zip LambdaFunctionOverHttps.py

Execute the following command.
aws configure
AWS Access Key ID [****************ULT3]: give your access ID
AWS Secret Access Key [****************FBXF]: give your secret access ID
Default region name [ap-northeast-2]: mention your region in which performing.
Default output format [None]: json
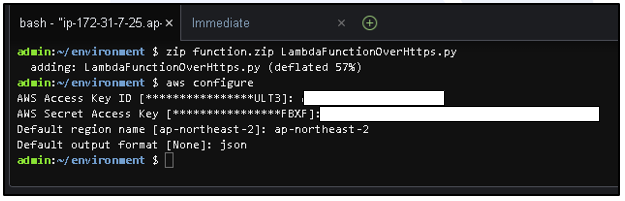
Create a Lambda function using the create-function AWS CLI command. For the role parameter, enter the execution role's Amazon Resource Name (ARN) that you copied earlier.
aws lambda create-function --function-name LambdaFunctionOverHttps \
--zip-file fileb://function.zip --handler LambdaFunctionOverHttps.handler --runtime python3.9 \
--role arn:aws:iam::123456789012:role/service-role/lambda-apigateway-role
Create a new file name input.txt and paste the following in the file.
{
"operation": "echo",
"payload": {
"somekey1": "somevalue1",
"somekey2": "somevalue2"
}
}
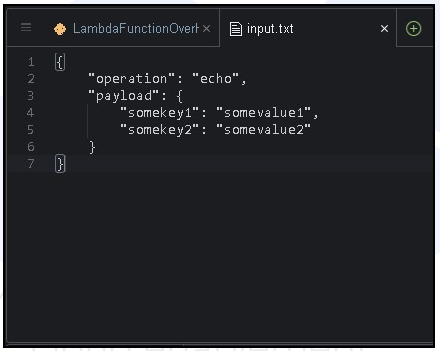
Execute the following command.
aws lambda invoke --function-name LambdaFunctionOverHttps \
--payload file://input.txt outputfile.txt --cli-binary-format raw-in-base64-out
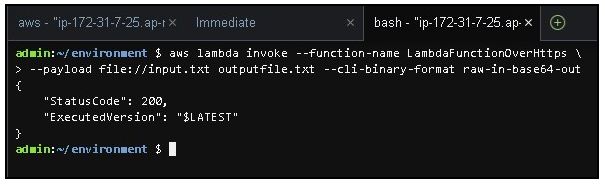
Confirm that your function performed the echo operation you specified in the JSON test event. Inspect the outputfile.txt file and verify it contains the following:
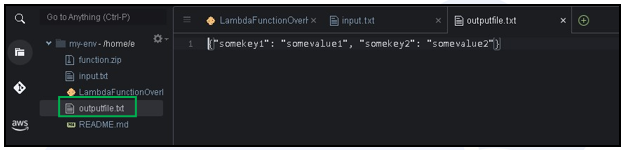
Step 4: In AWS management console, go to API gateway.
Choose Create API.
In the REST API box, choose Build.
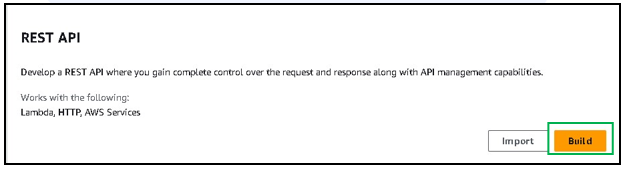
Under API details, leave New API selected, and for API Name, enter DynamoDBOperations.
Choose Create API.
On the Resources page for your API, choose Create Resource.
In Resource details, for Resource name enter DynamoDBManager.
Choose Create Resource.
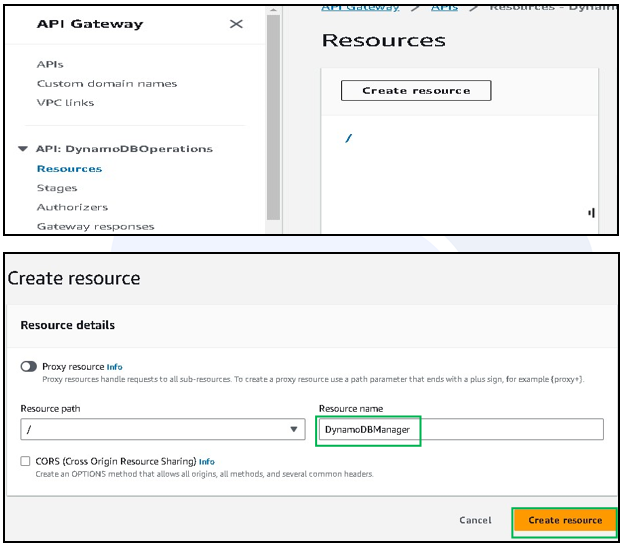
On the Resources page for your API, ensure that the /DynamoDBManager resource is highlighted. Then, in the Methods pane, choose Create Method.

For Method type, choose POST.
For Integration type, leave Lambda function selected.
For Lambda function, choose the Amazon Resource Name (ARN) for your function (LambdaFunctionOverHttps).
Choose Create method.
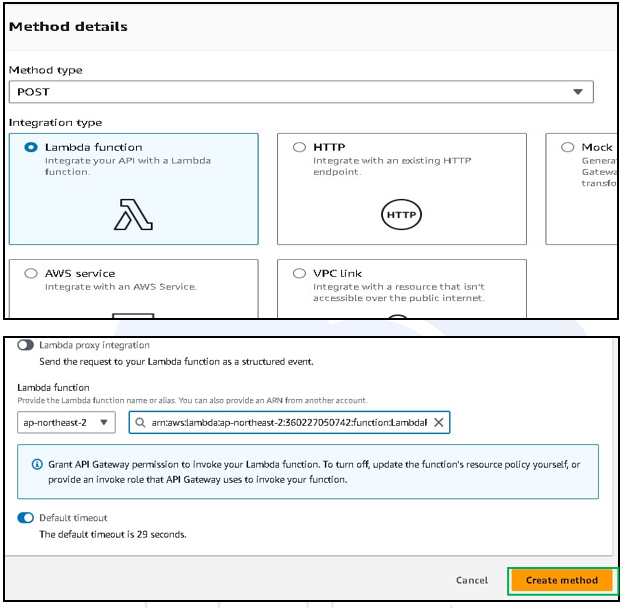
Step 5: In AWS management console, go to DynamoDB service.
Choose Create table.
Under Table details, do the following:
1. For Table name, enter lambda-apigateway.
2. For Partition key, enter id, and keep the data type set as String.
Under Table settings, keep the Default settings.
Choose Create table.

Step 6: In AWS management console, go to API Gateway service.
Choose the Test tab.
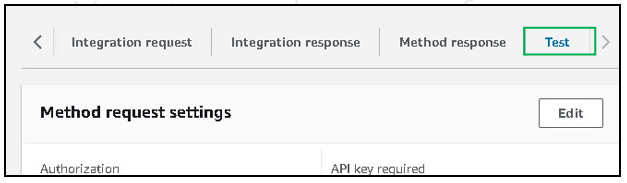
Under Test method, leave Query strings and Headers empty. For Request body, paste the following JSON:
{
"operation": "create",
"payload": {
"Item": {
"id": "1234ABCD",
"number": 5
}
}
}

Choose Test.
The results that are displayed when the test completes should show status 200. This status code indicates that the create operation was successful.

To confirm, check that your DynamoDB table now contains the new item.
Open the Tables page of the DynamoDB console and choose the lambda-apigateway table.
Chose Explore table items. In the Items returned pane, you should see one item with the id 1234ABCD and the number 5

Step 7: To update the item in your DynamoDB table
In the API Gateway console, return to your POST method's Test tab.
Under Test method, leave Query strings and Headersempty. For Request body, paste the following JSON:
{
"operation": "update",
"payload": {
"Key": {
"id": "1234ABCD"
},
"AttributeUpdates": {
"number": {
"Value": 10
}
}
}
}
3. Choose Test.
a. The results which are displayed when the test completes should show status 200. This status code indicates that the update operation was successful.
b. To confirm, check that the item in yout DynamoDB table has been modified.
Open the Tables page of the DynamoDB console and choose the lambda-apigateway table.
Chose Explore table items. In the Items returned pane, you should see one item with the id 1234ABCD and the number 10.

Step 8: Open the APIs page of the API Gateway console and choose the DynamoDBOperations API.
On the Resources page for your API choose Deploy API.

For Stage, choose *New stage*, then for Stage name, enter test.
Choose Deploy.

In the Stage details pane, copy the Invoke URL. You will use this in the next step to invoke your function using an HTTP request.

Go back to your Cloud9 environment and execute the following command in the terminal.
Run the following curl command using the invoke URL you copied in the previous step.
curl https://l8togsqxd8.execute-api.us-west-2.amazonaws.com/test/DynamoDBManager \
-d '{"operation": "create", "payload": {"Item": {"id": "5678EFGH", "number": 15}}}'

To verify that the create operation was successful, do the following:
1. Open the Tables page of the DynamoDB console and choose the lambda-apigateway table.
2. Choose Explore table items. In the Items returned pane, you should see an item with the id 5678EFGH and the number 15.
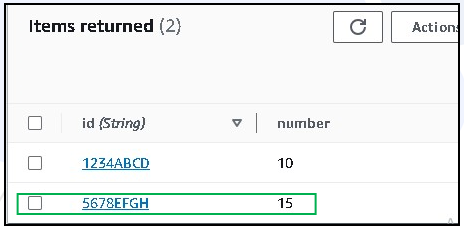
Run the following curl command to delete the item you just created. Use your own invoke URL.
curl https://l8togsqxd8.execute-api.us-west-2.amazonaws.com/test/DynamoDBManager \
-d '{"operation": "delete", "payload": {"Key": {"id": "5678EFGH"}}}'

Confirm that the delete operation was successful. In the Items returned pane of the DynamoDB console Explore items page, verify that the item with id 5678EFGH is no longer in the table.
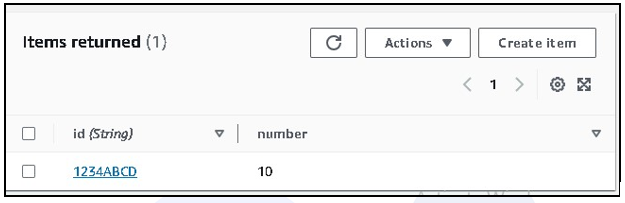
Note: Delete Cloud9 environment, DynamoDB Table, API and lambda function, if no longer needed.
Was this document helpful? How can we make this document better? Please provide your insights. You can download PDF version for reference.
We provide the best AWS training from Pune, India.
For AWS certification, contact us now.
Learnt to create a Microservice using AWS Lambda & Amazon API Gateway
Learnt to create a Microservice using AWS Lambda & Amazon API Gateway
Microservice using AWS lambda was explained clearly
It is very easy to understand the concept of microservices using AWS lambda
Easy to learn the concept of create a Microservice using AWS Lambda & Amazon API Gateway through this blog